[Quick Post] Smallest Print Shellcode
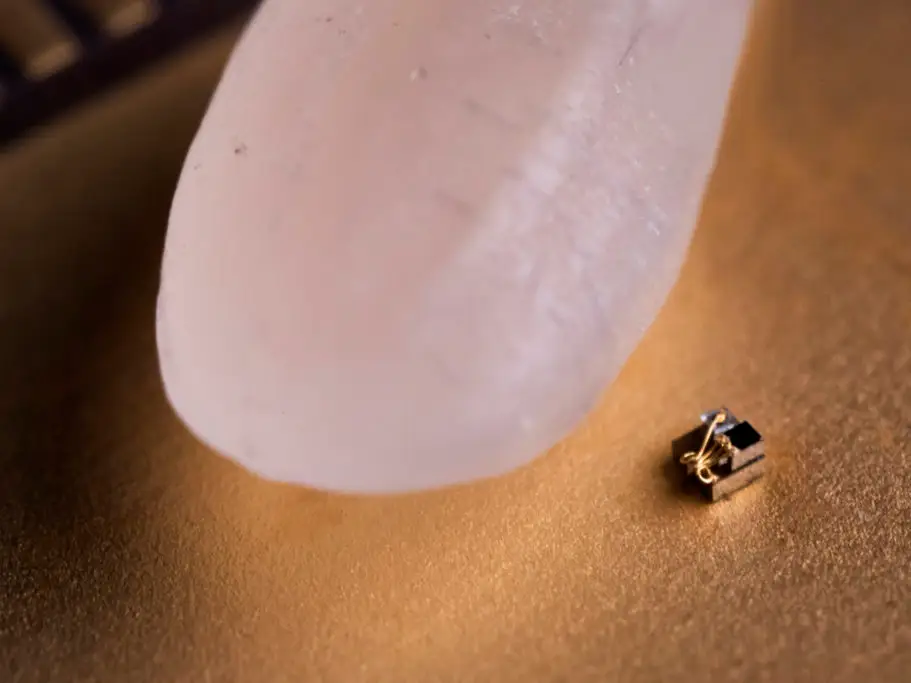
In this post, I will tell you about the smallest 32-bit Linux shellcode to write to stdout
with only syscalls and only using the .text
section.
So, I was doing Buffer Overflow CTF challenges from picoCTF 2022 and tried to achieve code execution primitive. I failed because challenges were compiled with -noexecstack
.
However, I gained some valuable knowledge and experience writing inline assembly.
Important details
I didn't need the program to exit properly after running the shellcode, so I avoided adding the corresponding syscall:
xor ebx, ebx
push 1
pop eax
int 80h
This section is only needed for NASM to properly assemble the code and isn't included in an actual binary file or shellcode.
global _start
section .text
_start:
Road to perfection
During my experiments, I needed the smallest possible shellcode to squeeze it into the buffer of a restricted size. I ended up with a simple shellcode that writes "Hi!" to stdout
:
global _start
section .text
_start:
mov DWORD [esp], "Hi!"
lea ecx, [esp] ; load address of the "Hi!" into ecx
mov edx, 3 ; move number of bytes to write into edx
mov ebx, 1 ; move file descriptor (1 - stdout) into ebx
mov eax, 4 ; move syscall number into eax
int 80h ; syscall
This shellcode is really small - just 27
bytes - \xc7\x04\x24\x48\x69\x21\x00\x8d\x0c\x24\xba\x03\x00\x00\x00\xbb\x01\x00\x00\x00\xb8\x04\x00\x00\x00\xcd\x80
.
However, we can make it smaller if we write only 1
byte to stdout
:
global _start
section .text
_start:
mov BYTE [esp], "H"
lea ecx, [esp]
mov edx, 1
mov ebx, 1
mov eax, 4
int 80h
Nice, now it's 24
bytes - \xc6\x04\x24\x48\x8d\x0c\x24\xba\x03\x00\x00\x00\xbb\x01\x00\x00\x00\xb8\x04\x00\x00\x00\xcd\x80
.
Wait, that's not the limit.
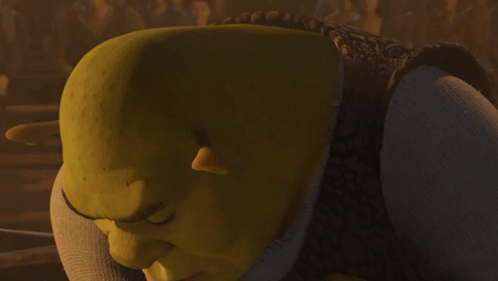
We can avoid those ugly 00
bytes by using push
/pop
instructions instead of mov
:
global _start
section .text
_start:
push "H"
lea ecx, [esp]
push 1
pop edx
push 1
pop ebx
push 4
pop eax
int 80h
Look at that beauty! Now, our shellcode is mere 16
bytes - \x6a\x48\x8d\x0c\x24\x6a\x01\x5a\x6a\x01\x5b\x6a\x04\x58\xcd\x80
.
This makes our shellcode the smallest possible one to write to stdout
.
Share your experience with shellcodes/inline assembly. See you soon!
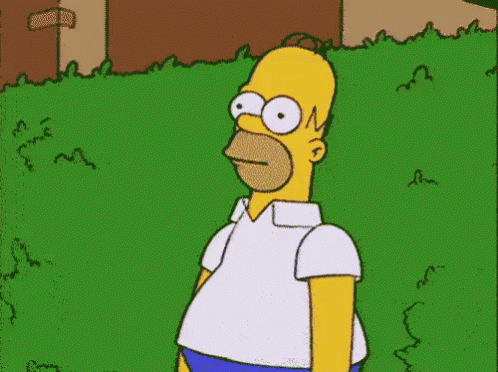
Time spent on preparing and publishing this post: 1h 20m.